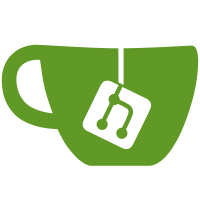
The script creates a Pelican file with Markdown metadata. It offers several options to adjut the values or the metadata.
94 lines
2.5 KiB
Bash
Executable file
94 lines
2.5 KiB
Bash
Executable file
#!/bin/bash
|
|
|
|
# A script to create journal entries
|
|
# Default behavior is to create an entry for the current date. See "help" for usage and options.
|
|
# Unsafe script
|
|
|
|
set -e
|
|
|
|
DATE=$(date +%Y-%m-%d)
|
|
AUTHOR="$WAREHOUSE_AUTHOR"
|
|
EDITMODE=0
|
|
|
|
print_help() {
|
|
echo "Usage: entry [OPTION…]"
|
|
echo " -a, --author author Change the author name in the created entry"
|
|
echo " -e, --edit Edit the entry file created using user defined editor. The editor is specified using the EDITOR environment variable. Does nothing if said variable is not defined"
|
|
echo " -h, --help Display this help message and exit."
|
|
echo " -n, --new [DATE] Create a new entry for the specified DATE (optional). If no date is specified, use current date. This is default behavior. DATE is expected in a format valid for the 'date' command"
|
|
}
|
|
|
|
while [ $# -gt 0 ]
|
|
do
|
|
case $1 in
|
|
-a|--author)
|
|
shift
|
|
if [ -z "$1" ]
|
|
then
|
|
echo "You must specify an author"
|
|
exit 1
|
|
fi
|
|
AUTHOR="$1"
|
|
shift
|
|
;;
|
|
-e|--edit)
|
|
EDITMODE=1
|
|
shift
|
|
;;
|
|
-h|--help)
|
|
shift
|
|
print_help
|
|
exit 0
|
|
;;
|
|
# Make a new template starting using current date (today)
|
|
-n|--new)
|
|
shift # past argument
|
|
if [ -n "$1" ]
|
|
then
|
|
echo "Date: $1"
|
|
DATE="$1"
|
|
shift
|
|
fi
|
|
;;
|
|
-*|--*)
|
|
echo "Unknown option $1"
|
|
exit 1
|
|
;;
|
|
*)
|
|
echo "Extra useless argument: $1"
|
|
exit 1
|
|
;;
|
|
esac
|
|
done
|
|
|
|
YEAR=$(date +%Y -d "$DATE")
|
|
MOUNTH=$(date +%B -d "$DATE")
|
|
DAY=$(date +%e -d "$DATE")
|
|
MOUNTHDIR=$(date "+%m" -d "$DATE")"-${MOUNTH^}"
|
|
FULLDATE="$(date +%e -d $DATE) $(date +%B -d $DATE) $(date +%Y -d $DATE)"
|
|
FILENAME="$DATE.md"
|
|
DIRPATH="content/journal/$YEAR/$MOUNTHDIR"
|
|
OUTPATH="$DIRPATH/$FILENAME"
|
|
|
|
if [ -f "$OUTPATH" ]
|
|
then
|
|
echo "Error, $OUTPATH: file exists"
|
|
exit 1
|
|
fi
|
|
if [ ! -d "$DIRPATH" ]
|
|
then
|
|
echo "Creating necessary directories $DIRPATH"
|
|
mkdir -p "$DIRPATH"
|
|
fi
|
|
echo "New template : $FULLDATE"
|
|
echo "Title: Journal du $DAY ${MOUNTH^} $YEAR" >> "$OUTPATH"
|
|
echo "Date: $DATE" >> "$OUTPATH"
|
|
echo "Author: $AUTHOR" >> "$OUTPATH"
|
|
echo "Category: $YEAR" >> "$OUTPATH"
|
|
echo "Tags:" >> "$OUTPATH"
|
|
echo "" >> "$OUTPATH"
|
|
|
|
if [ "$EDITMODE" -eq 1 ] && [ -n "$EDITOR" ]
|
|
then
|
|
$EDITOR "$OUTPATH"
|
|
fi
|